LeetCode 21. Merge Two Sorted Lists ( C ) – Easy
Posted On 2021 年 6 月 21 日
此題測驗的是鏈結串列 ( Linked List ) ,把兩個 Linked List 依照大小排序,串接在一起,並回傳串接完的 Linked List。
原文題目如下
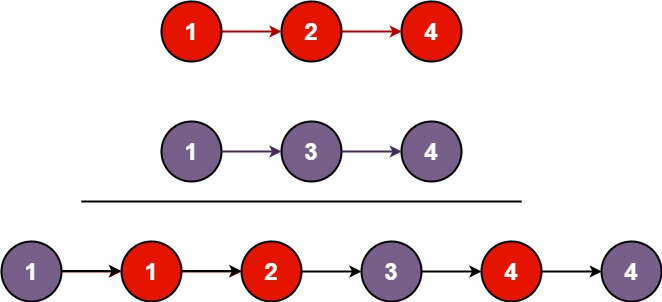
Merge two sorted linked lists and return it as a sorted list. The list should be made by splicing together the nodes of the first two lists.
Example 1:
Input: l1 = [1,2,4], l2 = [1,3,4]
Output: [1,1,2,3,4,4]
Example 2:
Input: l1 = [], l2 = []
Output: []
Example 3:
Input: l1 = [], l2 = [0]
Output: [0]
Constraints:
The number of nodes in both lists is in the range [0, 50].
-100 <= Node.val <= 100
Both l1 and l2 are sorted in non-decreasing order.
解題策略為透過 l1 儲存要回傳的 Linked List,每次迴圈依序比較 l1 跟 l2 的值,把 l2 的值依序連接到 l1 上,最後回傳 l1。
下面是我的程式碼
/** * Definition for singly-linked list. * struct ListNode { * int val; * struct ListNode *next; * }; */ struct ListNode* mergeTwoLists(struct ListNode* l1, struct ListNode* l2){ if(l1 == NULL) return l2; else if(l2 == NULL) return l1; else{ struct ListNode *ptr,*Fptr; ptr = l1; Fptr = ptr; while(l2!=NULL){ if(ptr->val > l2->val){ struct ListNode *temp = l2; l2 = temp->next; temp->next = ptr; if(ptr == l1){ l1 = temp; Fptr = l1; } else{ Fptr->next = temp; Fptr = temp; } } else{ if(ptr->next!=NULL){ if(Fptr == ptr){ } else{ Fptr = Fptr->next; } ptr = ptr->next; } else{ ptr->next = l2; l2 = NULL; } } } return l1; } }
下面是時間與空間之消耗
Runtime: 0 ms, faster than 100.00% of C online submissions for Merge Two Sorted Lists.
Memory Usage: 6 MB, less than 99.18% of C online submissions for Merge Two Sorted Lists.